ブログ/お知らせ Knowledge
【jquery】ユーザビリティをよくするサンプルコードまとめ
コーディング
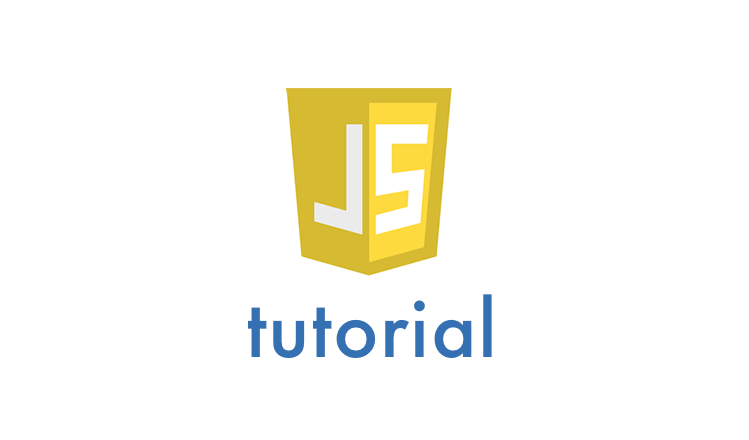
制作現場では決まって時間がない。
だから決まって使うソースコードは使いまわしたいですよね。
コピペで使える便利なコードを、役割別にまとめました。
今後もどんどん追加していくので、ブックマークとしておくと困った時に役に立つかも。
コードばかりでページが長くなってしまっているので、目次から先にチェックしてください。
目次
スムーススクロール
ページ内リンクをするするする、スムーススクロールの実装。
現場で絶対必要になるやつです。
// page scroll $(function () { // #で始まるアンカーをクリックした場合に処理 $('a[href^=#]').click(function () { // スクロールの速度 var speed = 400; // ミリ秒 // アンカーの値取得 var href = $(this).attr("href"); // 移動先を取得 var target = $(href == "#" || href == "" ? 'html' : href); // 移動先を数値で取得 var position = target.offset().top; // スムーススクロール $('body,html').animate({ scrollTop: position }, speed, 'swing'); return false; }); });
これだけ。
スティッキースクロール
これは.stickyという要素を通り過ぎた地点から、ページの上部で固定されて、以降付いてくるものです。
現場で追従するやつって呼ばれます。
.stickyがある地点に到達したと同時に、.is-fixedというclassを付与します。
最近ではCSSだけでできるようになりつつあるみたいで、早くIEブラウザ消えてなくならないかなって思っています。
<div class="l-header sticky"> <header class="header"> 固定したいグローバルナビとか </header> </div>
.sticky { box-shadow: 3px 5px 20px rgba(0, 0, 0, 0.05); background: #fff; &.is-fixed { top: 0; width: 100%; background: #fff; left: 0; position: fixed; z-index: 10000; } }
$(function () { var $fixElement = $('.sticky'); // 追従する要素 var baseFixPoint = $fixElement.offset().top; // 追従する要素の初期位置 var fixClass = 'is-fixed'; // 追従時に付与するclass // 要素が追従する処理 function fixFunction() { var windowScrolltop = $(window).scrollTop(); // スクロールが初期位置を通過しているとき if (windowScrolltop >= baseFixPoint) { $fixElement.addClass(fixClass); } else { $fixElement.removeClass(fixClass); } } $(window).on('load scroll', function () { fixFunction(); }); });
するするトップへ戻るボタン
$(function () { var topBtn = $('#back'); topBtn.click(function () { $('body,html').animate({ scrollTop: 0 }, 500); return false; }); });
スクロールすると現れて×で消せるバナー
<p class="season-banner"> <a href="#" target="_blank"> <img src="/dist/images/###.png" alt="###" /> </a> <i class="delete"> × </i> </p>
.season-banner { position: fixed; bottom: 20px; left: 0; width: 280px; img { width: 100%; position: relative; } i.delete { display: block; position: absolute; right: 0; top: 0; width: 25px; height: 25px; background: $base; text-align: center; font-size: 25px; line-height: 25px; &:hover { background: $black; color: #fff; } } }
$(function() { var topBtn = $('.season-banner'); var kesu = $(".delete"); $(topBtn,kesu).hide(); //スクロールが100に達したらボタン表示 $(window).scroll(function () { if ($(this).scrollTop() > 300) { //ボタンの表示方法 $(topBtn,kesu).fadeIn(); } else { //ボタンの非表示方法 $(topBtn,kesu).fadeOut(); } }); $(".delete").click(function() { $('.season-banner img ,.delete').hide(); }); });
Pjaxでページ遷移をシームレスにする
ページ遷移をスムーズにし、画面を切り替えずにページ遷移をする。
非同期通信と言われるやつです。
あまりにも切り替わるのが早いと、ページが切り替わったことさえわからなくなり、かえってユーザーを惑わせるので、アニメーションをつけると親切ですね。
参考:プログラムデザイナーへの道
まずはPjaxをダウンロードしてheadに記述しましょう。
http://falsandtru.github.io/pjax-api/
<header> ページ共通のヘッダーです </header> <section id="pjaxContent" class="pjax-test"> このなかが非同期通信で切り替わります。 この.btnのクラスをつけたものだけにPjaxが動作する。 </section> <footer> ページ共通のフッターです </footer>
//アニメーション用 $time: .5s; $ease: cubic-bezier(0.25, 0.46, 0.45, 0.94); #pjaxContent { position: relative; &.fadeIn { animation: fadeIn $time $easeOutCirc; } &.fadeOut { animation: fadeOut $time $easeOutCirc; } &.fadeOutDelay { animation: fadeOut $time .4s $easeOutCirc; } } @keyframes fadeIn { 0% { top: 100vh; transform: translate3d(20px, 0px, 100px); } 100% { top: 0; transform: translate3d(0px, 0px, 0px); } } @keyframes fadeOut { 0% { top: 0; } 100% { top: -100vh; } }
$(function(){ $.pjax({ area : '#pjaxContent', // 遷移するエリアを指定 link : '.btn', // イベント発火のターゲットを指定できる ajax: { timeout: 3000 }, // 読み込みにこれ以上かかる場合は通常遷移 wait : function(){// エフェクト分の待ち時間を作る(関数可) var wScr = $(window).scrollTop(); if(wScr){ return 800; }else{ return 400; } } }); $(document).on('pjax:fetch',function(){ // 消える時の処理 var wScr = $(window).scrollTop(); var doch = $(document).innerHeight(); //ページ全体の高さ var winh = $(window).innerHeight(); //ウィンドウの高さ var bottom = doch - winh; //ページ全体の高さ - ウィンドウの高さ = ページの最下部位置 if(wScr){ $('body,html').animate({bottom : 0},'100'); $('#pjaxContent').removeClass('fadeIn').addClass('fadeOutDelay'); }else{ $('#pjaxContent').removeClass('fadeIn').addClass('fadeOut'); } //直接書いてもよい // $('#pjaxContent').animate({ // 'left' : '-10px', // 'opacity' : 0 // }, 500); }); $(document).on('pjax:render',function(){ // 現れる時の処理 $('#pjaxContent').removeClass('fadeOut').addClass('fadeIn'); //直接書いてもよい // $('#pjaxContent').animate({ // 'left' : 0, // 'opacity' : 1 // }, 500); }); });
クラスで出し分けするグリッドレイアウト用SCSS
.l-grid { text-align: center; .l-grid-inner { font-size: 0; letter-spacing: 0; } .l-grid-item { display: inline-block; vertical-align: top; } //Modifier column &.l-grid-col2 { .l-grid-item { width: 50%; @include androidWidth(50%); } } &.l-grid-col3 { .l-grid-item { width: 33.3333%; } } &.l-grid-col4 { .l-grid-item { width: 25%; @include androidWidth(25%); } } &.l-grid-col5 { .l-grid-item { width: 20%; @include androidWidth(20%); } } &.l-grid-col6 { .l-grid-item { width: 16.6666%; } } /* Break point large */ @media all and (max-width: $break-lg) { &.l-grid-lg-col1 { .l-grid-item { width: 100%; } } &.l-grid-lg-col2 { .l-grid-item { width: 50%; @include androidWidth(50%); } } &.l-grid-lg-col3 { .l-grid-item { width: 33.3333%; } } &.l-grid-lg-col4 { .l-grid-item { width: 25%; @include androidWidth(25%); } } &.l-grid-lg-col5 { .l-grid-item { width: 20%; @include androidWidth(20%); } } &.l-grid-lg-col6 { .l-grid-item { width: 16.6666%; } } } /* Break point small */ @media all and (max-width: $break-sm) { &.l-grid-sm-col1 { .l-grid-item { width: 100%; } } &.l-grid-sm-col2 { .l-grid-item { width: 50%; @include androidWidth(50%); } } &.l-grid-sm-col3 { .l-grid-item { width: 33.3333%; } } &.l-grid-sm-col4 { .l-grid-item { width: 25%; @include androidWidth(25%); } } &.l-grid-sm-col5 { .l-grid-item { width: 20%; @include androidWidth(20%); } } &.l-grid-sm-col6 { .l-grid-item { width: 16.6666%; } } } }
イージング用の変数のSCSS
// Cubic $easeInCubic: cubic-bezier(0.550, 0.055, 0.675, 0.190); $easeOutCubic: cubic-bezier(0.215, 0.610, 0.355, 1.000); $easeInOutCubic: cubic-bezier(0.645, 0.045, 0.355, 1.000); // Circ $easeInCirc: cubic-bezier(0.600, 0.040, 0.980, 0.335); $easeOutCirc: cubic-bezier(0.075, 0.820, 0.165, 1.000); $easeInOutCirc: cubic-bezier(0.785, 0.135, 0.150, 0.860); // Expo $easeInExpo: cubic-bezier(0.950, 0.050, 0.795, 0.035); $easeOutExpo: cubic-bezier(0.190, 1.000, 0.220, 1.000); $easeInOutExpo: cubic-bezier(1.000, 0.000, 0.000, 1.000); // Quad $easeInQuad: cubic-bezier(0.550, 0.085, 0.680, 0.530); $easeOutQuad: cubic-bezier(0.250, 0.460, 0.450, 0.940); $easeInOutQuad: cubic-bezier(0.455, 0.030, 0.515, 0.955); // Quart $easeInQuart: cubic-bezier(0.895, 0.030, 0.685, 0.220); $easeOutQuart: cubic-bezier(0.165, 0.840, 0.440, 1.000); $easeInOutQuart: cubic-bezier(0.770, 0.000, 0.175, 1.000); // Quint $easeInQuint: cubic-bezier(0.755, 0.050, 0.855, 0.060); $easeOutQuint: cubic-bezier(0.230, 1.000, 0.320, 1.000); $easeInOutQuint: cubic-bezier(0.860, 0.000, 0.070, 1.000); // Sine $easeInSine: cubic-bezier(0.470, 0.000, 0.745, 0.715); $easeOutSine: cubic-bezier(0.390, 0.575, 0.565, 1.000); $easeInOutSine: cubic-bezier(0.445, 0.050, 0.550, 0.950); // Back $easeInBack: cubic-bezier(0.600, -0.280, 0.735, 0.045); $easeOutBack: cubic-bezier(0.175, 0.885, 0.320, 1.275); $easeInOutBack: cubic-bezier(0.680, -0.550, 0.265, 1.550);
まだまだ生きてるjquery
巷ではjavascriptのフレームワークとして、vue.jsが熱いみたいですね。
こちらを購入したので、どんどん僕も勉強します。
別々のファイルにして必要に応じて読み込むと使い勝手がいいです。
ほなね